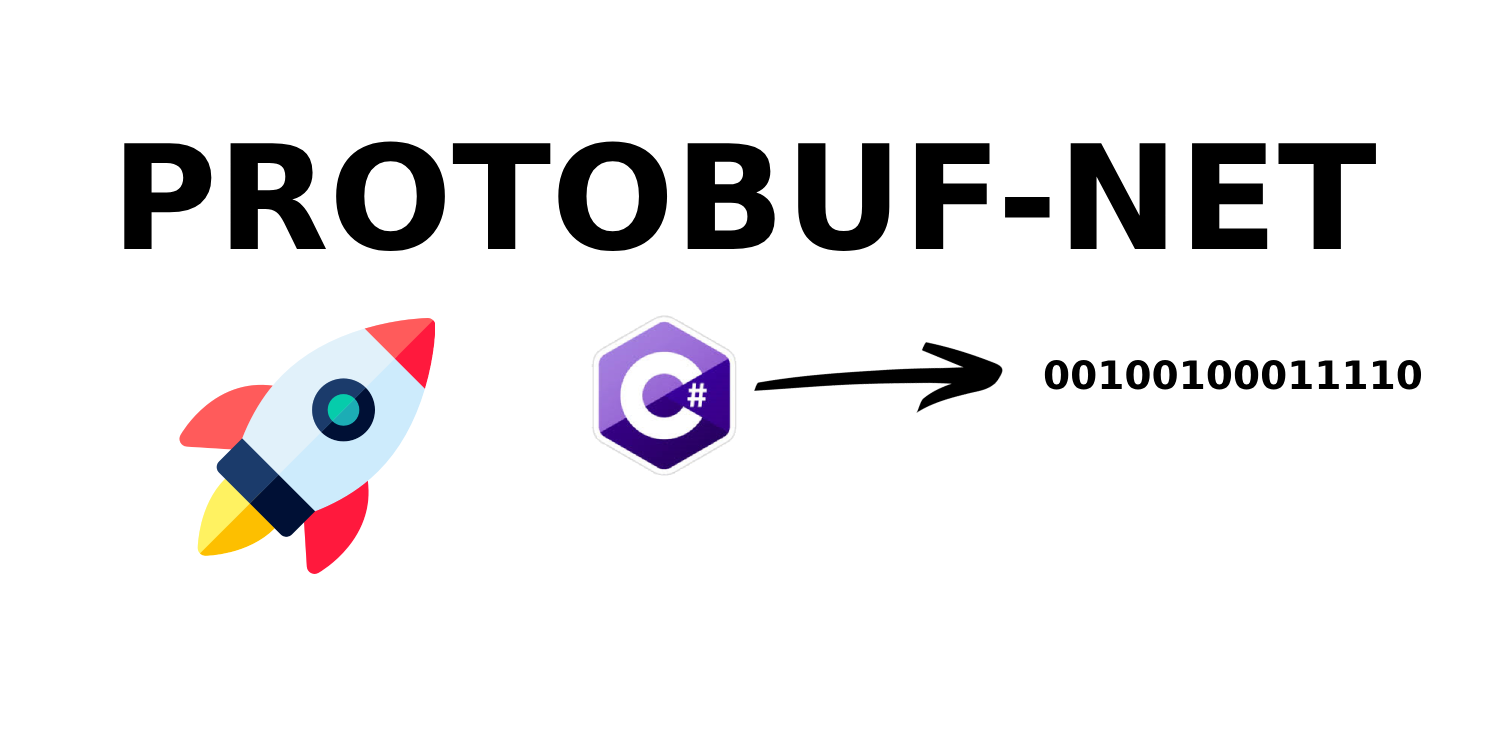
Publié le 17/02/2025
Par Christophe MOMMER
Data serialization is a major challenge for many applications, whether it's for inter-service communication, data storage, or performance optimization. Today, we're going to explore protobuf-net, a .NET library that enables you to use Protocol Buffers (or protobuf), a high-performance binary serialization format developed by Google.
Even though the JSON format currently dominates communications between services, the binary protobuf format can be extremely useful and practical for communication between different services within your infrastructure—especially for reducing the footprint of network transfers.
The library I'll be presenting is comprehensive and efficient, so this is just a brief introduction. For more details, please refer to its official repository.
The first step is quite straightforward: install the package (either through your IDE’s NuGet package manager or via the command line with dotnet add package protobuf-net
).
Let's take a very simple example that everyone can understand—the famous Person
class. The library automatically generates the proto contract information using attributes (with [ProtoContract]
above the class and [ProtoMember]
with the element’s position in the contract stream above the properties), for both serialization and deserialization:
using ProtoBuf;
[ProtoContract]
public class Person
{
[ProtoMember(1)]
public int Id { get; set; }
[ProtoMember(2)]
public string Name { get; set; }
[ProtoMember(3)]
public DateTime BirthDate { get; set; }
}
Once that's done, you simply use the Serializer
class from the package to perform serialization or deserialization operations:
Person person = new Person { Id = 1, Name = "Christophe Mommer", BirthDate = new DateTime(1988, 12, 18) };
using var stream = new MemoryStream();
Serializer.Serialize(stream, person);
var bytes = stream.ToArray();
The library is very easy to use, yet it’s also quite extensible and customizable for advanced use cases.
To compare the size of the protobuf stream to its JSON and XML equivalents, you get roughly the following sizes:
- XML = 252 bytes
- JSON = 69 bytes
- Protobuf = 26 bytes
As for performance:
In short, protobuf-net offers a great alternative for implementing communication in cases where it isn’t natively supported by gRPC.