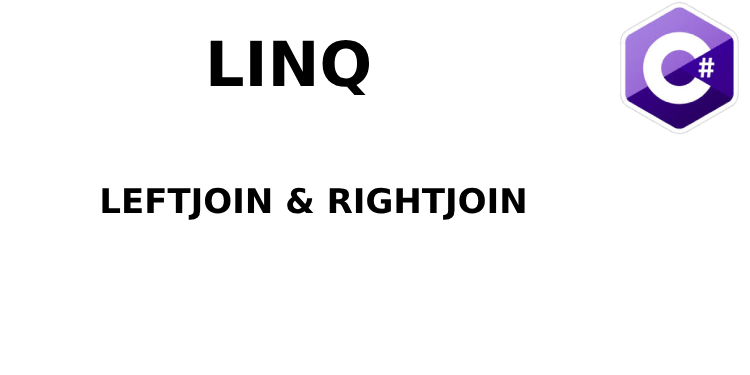
Published on 12/02/2025
By Christophe MOMMER
As with each new version of .NET, the framework’s creators treat us to new operators designed, on the one hand, to make our lives easier, and on the other, to give us even more possibilities — especially when using LINQ for SQL mapping with Entity Framework Core.
We already had the Join
operator, which lets us join collections of different objects based on a common criterion and obtain a result projection that includes only the elements satisfying the join condition (equivalent to an INNER JOIN
in SQL).
However, there were still some cases that weren’t covered, particularly when we want to retrieve, as in SQL, all objects from the “left or right” collection.
Let’s take a simple example:
lass Person
{
public int Id { get; set; }
public string Name { get; set; }
}
class Address
{
public int PersonId { get; set; }
public string City { get; set; }
}
List<Person> people =
[
new Person { Id = 1, Name = "Alice" },
new Person { Id = 2, Name = "Bob" },
new Person { Id = 3, Name = "Charlie" }
];
List<Address> addresses =
[
new Address { PersonId = 1, City = "Paris" },
new Address { PersonId = 2, City = "Lyon" },
new Address { PersonId = 4, City = "Bordeaux" }
];
In this code snippet, it’s clear that each person does not necessarily have an address. Therefore, if we performed a Join
on these two collections, Charlie would not appear in the result. Conversely, the person with ID 4 obviously wasn’t loaded, so their address wouldn’t appear either. Thanks to these new operators, we can now choose to retrieve them anyway.
For example, if I want to retrieve all people and, for those who don’t have an address, display “Unknown” I need to perform a join on the person’s ID while treating the people collection as the main table (i.e., a left join):
var leftJoinResult = people.LeftJoin(
addresses,
person => person.Id,
address => address.PersonId,
(person, address) => new
{
person.Name,
City = address?.City ?? "Unknown"
});
foreach (var item in leftJoinResult)
{
Console.WriteLine($"{item.Name} lives in {item.City}");
}
Of course, the same principle applies to RightJoin
, except that this time, the collection passed as a parameter is considered the main one. And naturally, since we’re only in the early preview stages, there’s a good chance that these new operators will eventually be supported by EF Core for the equivalent SQL operations.
A welcome addition to the framework!